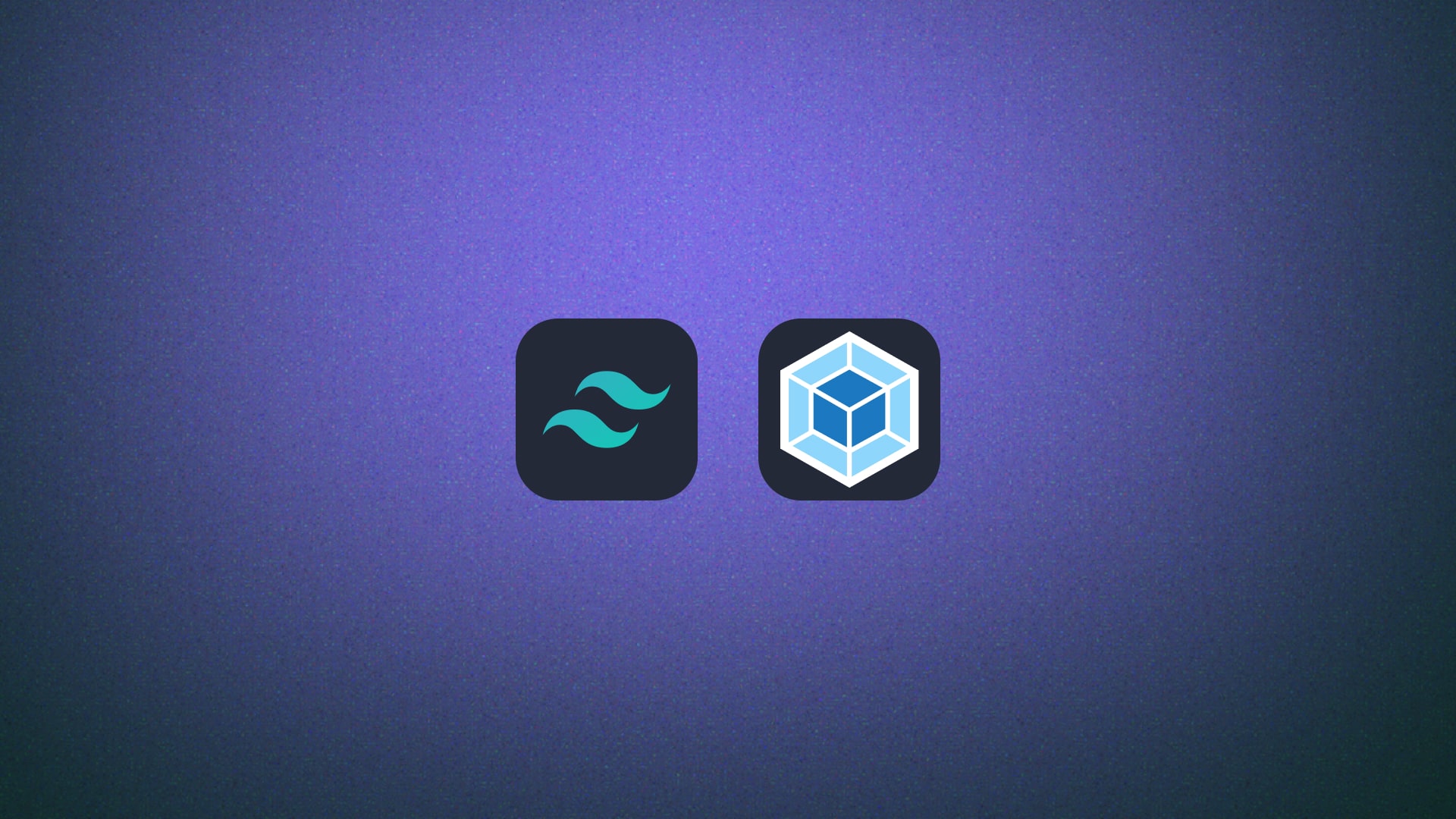
Tailwind CSS is a utility-first CSS framework that has gained immense popularity due to its flexibility and rapid development capabilities. In this tutorial, we’ll walk through the process of integrating Tailwind CSS into a project using Webpack as the build tool. This setup will allow you to leverage Tailwind’s powerful utility classes while benefiting from Webpack’s module bundling capabilities.
Introduction
Hello again, React enthusiasts! You’ve reached the third milestone in our four-part series on setting up the the codebase for crafting professional-grade React applications. It’s time to add some style to our well-structured, thoroughly tested project. Here’s where we stand in our journey:
- Setting Up a React Project with TypeScript and Webpack: A Step-by-Step Guide
- Easily Setup Test Driven Development with React Testing Library and Jest
- How to Set Up Tailwind CSS with Webpack: A Step-by-Step Guide (You are here)
- Mastering Dynamic Theming in Tailwind CSS: A Step-by-Step Guide
In this third installment, we’re focusing on integrating Tailwind CSS into your Webpack-powered React project. Tailwind CSS has revolutionized the way developers approach styling, and we’re going to show you how to harness its power. You’ll learn how to configure Webpack to work seamlessly with Tailwind, enabling you to rapidly build custom, responsive designs without leaving your JSX. By the end of this guide, you’ll have a turbocharged styling workflow that will make creating beautiful UIs a breeze. Ready to give your React app a stylish makeover? Let’s dive in!
Step 1: Install Tailwind CSS and Its Dependencies
First, we need to install Tailwind CSS and its peer dependencies. Open your terminal and run the following command:
npm i -D tailwindcss postcss autoprefixer
This command installs Tailwind CSS, PostCSS (a tool for transforming CSS with JavaScript), and Autoprefixer (a PostCSS plugin to parse CSS and add vendor prefixes to CSS rules) as development dependencies.
Next, create a Tailwind configuration file:
npx tailwindcss init
This command generates a tailwind.config.js
file in your project root, which you’ll use to customize your Tailwind installation.
Step 2: Install CSS Loaders for Webpack
Webpack needs specific loaders to process CSS files. Install these loaders by running:
npm i -D style-loader css-loader postcss-loader postcss-preset-env
These loaders will help Webpack understand and process CSS files:
Option | Purpose |
---|---|
style-loader | Injects CSS into the DOM. |
css-loader | Interprets @import and url() like import/require() and resolves them. |
postcss-loader | Processes CSS with PostCSS. |
postcss-preset-env | Converts modern CSS into something most browsers can understand. |
Step 3: Configure Webpack for CSS Files
Now, we need to tell Webpack how to handle CSS files. Add the following configuration to your webpack.config.js
file:
module: {
rules: [
{
test: /\.css$/i,
include: path.resolve(__dirname, "src"),
use: ["style-loader", "css-loader", "postcss-loader"],
},
],
},
This configuration tells Webpack to use the installed loaders for any CSS file within the src
directory. The loaders are applied in reverse order: first postcss-loader
, then css-loader
, and finally style-loader
.
Step 4: Configure PostCSS
Create a postcss.config.js
file in your project root and add the following content:
module.exports = {
plugins: {
tailwindcss: {},
autoprefixer: {},
}
}
This configuration tells PostCSS to use Tailwind CSS and Autoprefixer plugins when processing your CSS.
Step 5: Configure Tailwind CSS
Open the tailwind.config.js
file created in Step 1 and update it to include the paths to your template files:
/** @type {import('tailwindcss').Config} */
module.exports = {
content: ["./src/**/*.{html,js,jsx,ts,tsx}"],
theme: {
extend: {},
},
plugins: [],
}
This configuration tells Tailwind which files to scan for class names to include in your CSS. Adjust the paths according to your project structure.
Step 6: Add Tailwind Directives to Your CSS
Create a main CSS file (e.g., src/styles.css
) and add the following Tailwind directives:
@tailwind base;
@tailwind components;
@tailwind utilities;
These directives inject Tailwind’s base styles, component classes, and utility classes into your CSS.
Step 7: Start Your Build Process
Finally, start your development server or build process. The exact command depends on your package.json
configuration, but it’s typically something like:
npm run dev
This command will start Webpack, which will now process your CSS files using Tailwind CSS.
Conclusion
Congratulations on successfully integrating Tailwind CSS into your Webpack-powered React project! You’ve now unlocked a world of rapid UI development with Tailwind’s utility-first approach. This setup allows you to leverage the full power of Tailwind’s customization options while maintaining the benefits of Webpack’s module bundling.
As you start using Tailwind in your components, remember to leverage its responsive design features and explore the @apply
directive for creating reusable utility combinations. Don’t be afraid to customize the tailwind.config.js
file to align the framework with your project’s specific design needs.
In the final part of our series, we’ll take your Tailwind skills to the next level by implementing dynamic theming. You’ll learn how to create a flexible color scheme that can adapt on the fly, providing a personalized experience for your users. Stay tuned to master the art of dynamic styling in React!